JavaScript Game Development

Akari
Akari is mouse or touch screen controlled - no keysPerformance fixes
A couple of serious issues have been addressed that were crippling the game's performance.
I need to research why the following features were impacting the frame rate so much but here are the offending functions:
g.ctx.globalCompositeOperation="destination-out";
g.ctx.globalAlpha = 0.3;
The namespace g{}
is a global object that I use to store much of the game's structure. ctx
is the canvas context as defined:
g.canvas = document.querySelector('canvas');
g.ctx = g.canvas.getContext('2d');
According to Mozilla's own documentation the globalCompositeOperation is fully supported in all major browsers yet my own tests for this show a serious performance hit in all of them.
I can only deduce that it is because the game is attempting to perform this operation up to 60 times a second courtesy of requestAnimationFrame()
. But the reality is that I just don't know.
Another expensive operation was the shadowing afforded by the canvas API.
g.ctx.shadowColor = "#000000";
g.ctx.shadowOffsetX = 0;
g.ctx.shadowOffsetY = 2;
g.ctx.shadowBlur = 0;
I need to do more testing of this but I suspect that the shadowBlur
call is the offender.
Either way, the effect added little to the experience so I fully removed it.
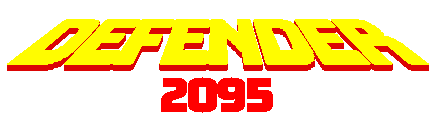
Defender 2095 (in development)
Player's ship changing direction
I have a few issues with the way the player's ship changes direction.
In the original Defender the ship simply switched to face in the opposite direction and the ground and player ship shifted accordingly until the ship was in the exact opposite x co-ordinate.
The way I have it at the moment isn't quite right and the ship 'swings' far too much. Needs some closer attention as currently it's fairly maddening to 'swing' and reverse into an alien that you had no idea was there.
Update: resolved the player ship shifting issue and added a small shift for aesthetics. Finally feel that controlling the ship is fun.
Dev log - Development started January 2023
- Implemented the (bastard) saucer
- Added the scanner with some rudimentary percentage calculations
- Need to attach human to player ship when caught while falling
- Create x index beneath ship to allow more than one human to be collected
- Create 'rescued' flag for the human item when collected by the player's ship
- Need to destroy humans if they fall from a great height (ground - 300px)
- Issue with aliens clinging to player when in mutant mode - this also stops the drawing of stars and cancels effective movement of other sprites!
- Issue with humans not being properly released in some situations
- Aliens becoming mutants if they successfully pull a human to the top of the screen (need to create Mutant graphic)
- Aliens now taking humans and dropping them when destroyed
- Removed explosions and now use spawnSparks() on alien and player death.
- playerAlienCollision() now triggering playerDeath()
- Abandoned the DropZone graphics. Implemented a classic Defender look and feel with the 'vector' landscape and green aliens.
- Implemented the classic Defender death cycle - flashing white screen then explosion.
- Playship now drifts back to origin X when not thrusting.
- Ripped some original Defender sound effects.
- Enhanced player ship movement based on velocity (g.groundspeed).
- Improved ship 'swing' when changing directions.
- Increased alien Y threshold to allow them to reach the floor and interact with humans.
- Abandoned the concept of 'thrust' and opted for a constantly moving terrain. The original Defender was a spaghetti-fingered affair and I wanted to avoid that.
- Implemented a Dropzone (from Atari 800XL) feel to the background graphics. Drew the art in Photoshop at half scale (480 x 98) and exported for web at 200% scale using nearest neighbour to present the pixelated look.
- Fixed backdrop 'tearing' when it reaches the screen bounds and gets re-drawn at the other side.
- Simply re-drawing the backdrop at object.width * -1 for backdrops travelling left to right, or at object.width (essentially the canvas width) for backdrops travelling right to left, wasn't good enough. I needed to calculate precisely how far below zero or beyond canvas width the object had been drawn and then add or subtract that difference to / form the new x co-ordinate). Otherwise the graphics would develop gaps and appear to 'tear'. The reason for this precision is that as x is adjusted on movement I take into consideration the DELTA time and multiply the x,y values by that.
- Ripped the codebase for Space Invaders 2095 and created a horizontal scrolling backdrop.
- Figuring out how to create the authentic Defender lasers.
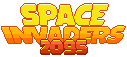
Space Invaders 2095 - development completed January 2023
Dev log - Development started December 2022
- May 2023 - modifications
- Added touch control - can now be played using a mobile phone / tablet etc.
- Removed the high score table for touch control - too much faff for selecting the characters when entering your initials
- Touch control uses localStorage for saving the high score
- Lightened the playership's colour to avoid clash with environment - better for mobile play
- Raised the start height of the playership to better accommodate touch movement and reduce clash with browser interface
- Fixed incorrect call to spawnExplosionXY() on playerdeath.
- Set base speed of player (m.player.speed) to 6 instead of 4. Give the player a fighting chance!
- Removed key repeat and the burst of lasers.
- Throttled player lives count on each game tick to overcome browser config to provide user defined lives count.
- Added a couple of new alien formations.
- Sorted the colour cycling on the hi score table. (Still not 100% happy with it but it will do)
- Adjusted the canvas width and height variables to better suit landscape orientation.
- Re-positioned hiscore input characters and player cursor to fit new orientation parameters.
- Re-adjusted the in-game sprite x,y of various items to be calculated rather than hard coded.
- Fixed a glitch in the explosion rendering. Animation frame was being reset in the wrong location causing a flicker of the first frame at the end of the animation sequence.
- Ditched the idea of presenting an 80s style arcade cabinet backdrop.
- Created 3 different saucers each with their own powerup drop: Lasers, Speed Boost, Extra Life.